Chapter 0: Introduction and Setup
MongoDB URI
Given the following MongoDB URI:
mongodb+srv://brigitte:bardot@xyz-1234.srv.net/admin
which of the following statements is true ?
- The password used to authenticate is bardot
Chapter 0: Introduction and Setup
Setting Up Atlas
mongorestore --gzip --archive=sampledata.archive.gz
https://www.mongodb.com/docs/database-tools/mongorestore/
mongorestore — MongoDB Database Tools
Docs Home → MongoDB Database ToolsThe mongorestore program loads data from either a binary database dump created by mongodump or the standard input into a mongod or mongos instance.Run mongorestore from the system command line, not the mongo shell.See al
www.mongodb.com
https://www.mongodb.com/try?jmp=university#compass
Try MongoDB Atlas Products
Try MongoDB Atlas products free. Developers can choose to use in the cloud or download locally. Either way, our software makes it easy to work with data.
www.mongodb.com
https://www.mongodb.com/docs/compass/master/aggregation-pipeline-builder/
Aggregation Pipeline Builder — MongoDB Compass
Docs Home → MongoDB CompassNew in version 1.14.0The Aggregation Pipeline Builder in MongoDB Compass provides the ability to create aggregation pipelines to process data. In aggregation pipelines, documents in a collection or view pass through stages wher
www.mongodb.com
https://www.mongodb.com/docs/compass/master/export-pipeline-to-language/
Export Pipeline to Specific Language — MongoDB Compass
Docs Home → MongoDB CompassNew in version 1.15.0You can use the Aggregation Pipeline Builder to export your finished pipeline to one of the supported languages; Java, Node, C#, and Python 3. This feature facilitates formatting and exporting pipelines cre
www.mongodb.com
Chapter 0: Introduction and Setup
Lab (Ungraded): Create or Reuse Atlas Cluster
Chapter 0: Introduction and Setup
OVERVIEW
Chapter 0: Introduction and Setup
README: Setting Up mflix
# navigate to the mflix-python directory
cd mflix-python
# enable the "conda" command in Terminal
echo ". /anaconda3/etc/profile.d/conda.sh" >> ~/.bash_profile
source ~/.bash_profile
# create a new environment for MFlix
conda create --name mflix
# activate the environment
conda activate mflix
pip install -r requirements.txt
mv dotini_unix .ini # on Unix
rename dotini_win .ini # on Windows
from bson.py3compat import abc, string_type, PY3, text_type
ImportError: cannot import name 'abc' from 'bson.py3compat' (C:\Users\formin\AppData\Local\Programs\Python\Python39\lib\site-packages\bson\py3compat.py)
-> pip uninstall bson
-> uninstall pymongo
-> pip install pymongo
pytest -m connection
Chapter 0: Introduction and Setup
Ticket: Connection
python run.py
Now proceed to the status page to run the full suite of integration tests and get your validation code.
After passing the relevant tests, what is the validation code for Connection?
-> 5a9026003a466d5ac6497a9d
Chapter 1: Driver Setup
Introduction to Chapter 1
Chapter 1: Driver Setup
MFlix Application Architecture
During this course, which of the following files in mflix will you have to edit?
-> db.py
Chapter 1: Driver Setup
MongoClient
Chapter 1: Driver Setup
Basic Reads
What do the methods find() and find_one() have in common?
- They are used to query documents in a collection.
- They accept a query predicate.
- They accept a field projection.
User Story
"As a user, I'd like to be able to search movies by country and see a list of movie titles. I should be able to specify a comma-separated list of countries to search multiple countries."
Task
Implement the get_movies_by_country method in db.py to search movies by country and use projection to return the title and _id field. The _id field will be returned by default.
You can find examples in notebooks/your_first_read.ipynb.
MFlix Functionality
Once you complete this ticket, the UI will allow movie searches by one or more countries.
Testing and Running the Application
Make sure to look at the tests in test_projection.py to understand what is expected.
You can run the unit tests for this ticket by running:
pytest -m projection
Once the unit tests are passing, run the application with:
python run.py
Now proceed to the status page to run the full suite of integration tests and get your validation code.
After passing the relevant unit tests, what is the validation code for Projection?
def get_movies_by_country(countries):
"""
Finds and returns movies by country.
Returns a list of dictionaries, each dictionary contains a title and an _id.
"""
try:
"""
Ticket: Projection
Write a query that matches movies with the countries in the "countries"
list, but only returns the title and _id of each movie.
Remember that in MongoDB, the $in operator can be used with a list to
match one or more values of a specific field.
"""
# TODO: Projection
# Find movies matching the "countries" list, but only return the title
# and _id. Do not include a limit in your own implementation, it is
# included here to avoid sending 46000 documents down the wire.
#return list(db.movies.find().limit(1))
return list(db.movies.find({"countries": {"$in": countries}},{"title":1}))
except Exception as e:
return e
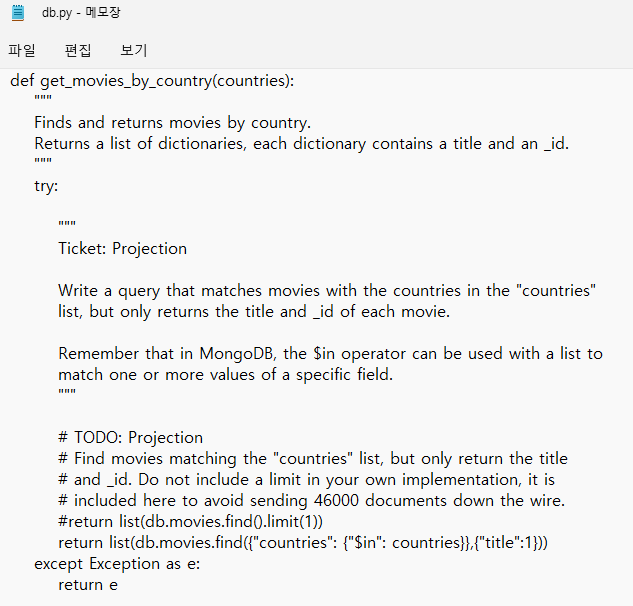
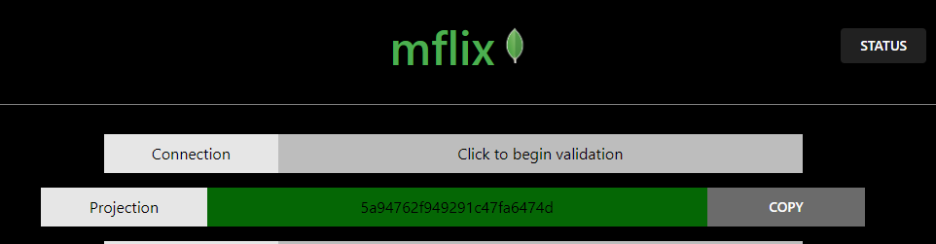
-> 5a94762f949291c47fa6474d
Chapter 1: Driver Setup
Ticket: Text and Subfield Search
User Story
"As a user, I'd like to be able to search movies by cast members, genre, or perform a text search of the plot summary, full plot, and title."
Task
For this ticket, you will need to modify the method build_query_sort_project in db.py to allow the following movie search criteria:
genres: finds movies that include any of the wanted genres.
Already, the build_query_sort_project method is able to return results for two different types of movie search criteria:
text: performs a text search in the movies collection
cast: finds movies that include any of the wanted cast
You just need to construct the query that queries the movies collection by the genres field.
Hint
Check the implementation of similar formats of search criteria - the genres query should be similar.
MFlix Functionality
Once you complete this ticket, the UI will allow movie searches by members of the cast, movie genres, movie title, and plot summary.
A text index was created for you when you restored the collections with mongorestore, so these queries will be performant once they are implemented.
Testing and Running the Application
Make sure to look at the tests in test_text_and_subfield_search.py to understand what is expected.
You can run the unit tests for this ticket with:
pytest -m text_and_subfield_search
Once the unit tests are passing, run the application with:
python run.py
Now proceed to the status page to run the full suite of integration tests and get your validation code.
After passing the relevant tests, what is the validation code for Text and Subfield Search?
def build_query_sort_project(filters):
"""
Builds the `query` predicate, `sort` and `projection` attributes for a given
filters dictionary.
"""
query = {}
# The field "tomatoes.viewer.numReviews" only exists in the movies we want
# to display on the front page of MFlix, because they are famous or
# aesthetically pleasing. When we sort on it, the movies containing this
# field will be displayed at the top of the page.
sort = [("tomatoes.viewer.numReviews", DESCENDING), ("_id", ASCENDING)]
project = None
if filters:
if "text" in filters:
query = {"$text": {"$search": filters["text"]}}
meta_score = {"$meta": "textScore"}
sort = [("score", meta_score)]
project = {"score": meta_score}
elif "cast" in filters:
query = {"cast": {"$in": filters["cast"]}}
elif "genres" in filters:
"""
Ticket: Text and Subfield Search
Given a genre in the "filters" object, construct a query that
searches MongoDB for movies with that genre.
"""
# TODO: Text and Subfield Search
# Construct a query that will search for the chosen genre.
#query = {}
query = {"genres": {"$in": filters["genres"]}}
return query, sort, project
def get_movies(filters, page, movies_per_page):
"""
Returns a cursor to a list of movie documents.
Based on the page number and the number of movies per page, the result may
be skipped and limited.
The `filters` from the API are passed to the `build_query_sort_project`
method, which constructs a query, sort, and projection, and then that query
is executed by this method (`get_movies`).
Returns 2 elements in a tuple: (movies, total_num_movies)
"""
query, sort, project = build_query_sort_project(filters)
if project:
cursor = db.movies.find(query, project).sort(sort)
else:
cursor = db.movies.find(query).sort(sort)
total_num_movies = 0
if page == 0:
total_num_movies = db.movies.count_documents(query)
"""
Ticket: Paging
Before this method returns back to the API, use the "movies_per_page"
argument to decide how many movies get displayed per page. The "page"
argument will decide which page
Paging can be implemented by using the skip() and limit() methods against
the Pymongo cursor.
"""
# TODO: Paging
# Use the cursor to only return the movies that belong on the current page.
#movies = cursor.limit(movies_per_page)
if page == 0:
movies = cursor.limit(movies_per_page)
else:
movies = cursor.skip(int(page) * int(movies_per_page)).limit(movies_per_page)
return (list(movies), total_num_movies)
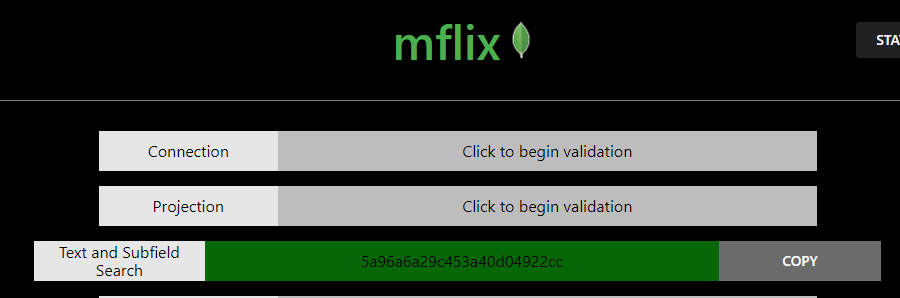
-> 5a96a6a29c453a40d04922cc
'푸닥거리' 카테고리의 다른 글
OOP(Object-Oriented Programming) 의 SOLID 원칙 (0) | 2022.07.10 |
---|---|
아키텍처 패턴(Architecture Patterns), 디자인 패턴(Design Pattern) (0) | 2022.07.09 |
M121 The MongoDB Aggregation Framework (0) | 2022.06.18 |
InnoDBClusterSet (0) | 2022.06.16 |
외부화를 위한 Amazon의 선언 (0) | 2022.06.13 |
댓글